Web development
How to Build a Web Application: Web Development Then and Now

Rano Salieva
February 28, 2025
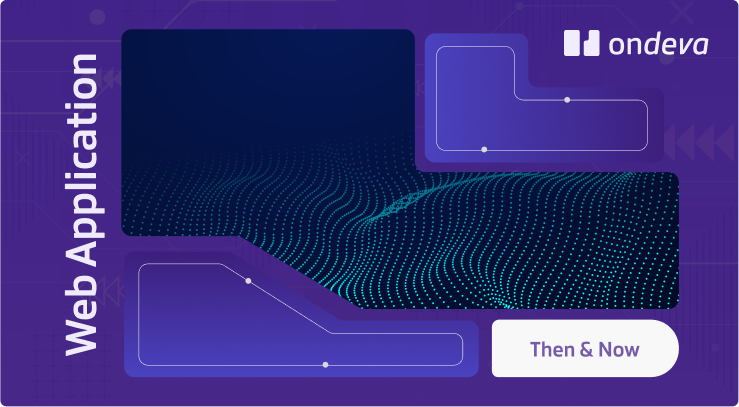
In a nutshell
This article shows you how to build a simple web app. It is intended for beginner developers, or anyone interested in web application development.
We cover the following topics:
- What are web applications, and how are they different from mobile applications?
- History of web application development and how it evolved from traditional to modern (low-code/no-code) approach.
- The architecture of the web app and how each part is designed and built.
- We explore some basic developer concepts and design patterns supporting web application development.
- You will learn the process, tools, and exact steps for creating a full-stack web application.
What is a web application?
The defining feature of web applications is that they can be accessed through the Web or the Internet. The first web applications were based on static web pages. Later, interactivity and intelligent user interactions were added, such as recommender systems or semantic web search.
So, a web application is characterized by the following:
- It uses the web, supported by JavaScript, JSON, XML, cloud computing, web interoperability (REST), etc.
- Has a client-service architecture with a network in between.
- Leverages Web - a system of connected web pages containing media and hyperlinks.
Web applications architecture includes:
- The presentation layer, which represents a user interface
- The data layer, where data is manipulated and modeled
- The workflow layer is where workflows and business logic are built.
Building a web application - How do you start?
Before building a web application, you must decide and configure your development environment.
The development environment is the tools, languages, frameworks, and applications you will use to build an app.
These days, many developers turn to low-code tools to build web applications. Low-code platforms like ondeva offer developers a ready-to-go environment to build fully functional web apps without code.
Backend languages and frameworks
Frameworks are software environments with pre-defined and customizable components that speed up development. It is rare to create an app from scratch without a framework.
Examples of frameworks include Ruby on Rails (Ruby), Play (Java/Scala), Django (Python), Symfony (PHP), and Sails.js (Node.js). Your device may have pre-loaded backend frameworks, but you must check the version.
Front-end frameworks and languages
Front-end constructs the presentation layer with which users see and interact. These languages include CSS, HTML, and JavaScript. While core languages like HTML, CSS, and JavaScript are available in a web browser, some frameworks and libraries must be pre-loaded.
Database and database management tools
The database will store all application data. The choice of the database depends on your project requirements (MySQL, PostgreSQL, MongoDB). You need to install a database management system. SQLite can be a good choice for beginners and doesn’t require installation.
Development tools and environments
These are tools that support standard development practices. They include code editors (Sublime), version control systems (Git), and web developer tools (browser-specific). Some programmers prefer to use IDEs instead of the three tools. IDEs combine a database browser, version control tool, and code editor in one environment. WebStorm is an example of an IDE.
Development process
These practices, for example Agile, organize programming and development to increase productivity and quality.
Middleware frameworks
Middleware allows the client and server to interact by intercepting HTTP requests and responses and enabling you to modify them. The choice of middleware depends on the choice of a backend framework—Express.js Middleware for Node.js and Rack for Ruby on Rails.
You can substitute some parts of the development environment and process with platforms and tools that simplify development, such as Backend-as-a-Service or Platform-as-a-Service. These platforms offer pre-built, configurable services, which speed up development and save costs.
Another option is a low-code platform like ondeva, that provides a visual interface to build full-stack applications. You can alternate between different layers of the app, from backend data and logic to frontend design, without losing any progress. With version control, you can see changes live and revert if needed. While easy to learn and use, ondeva still allows for significant customization and scalability.
Looking to develop a web application rapidly?
As a low-code platform built by developers, ondeva helps you focus on creative tasks.
Start for free. Pay only after 100 monthly page views.
Sign-up and get building in seconds - no payment, credit card, or demo required.
Mobile app development vs Web development
This article will show how to build a simple web application. Web applications are very different from mobile applications.
Web apps can also function like mobile apps. These web apps are designed to bring users both web and mobile app experiences. They fit different screen sizes, can work offline, and send push notifications. This approach (Progressive Web Apps) allows developers to build a single version of an application for web and mobile platforms.

Mobile apps
Are platform-dependent and specifically developed for iOS or Android. They can be accessed offline and are distributed through app stores like Google Play or the App Store. They usually require app store approval before release.

Web apps
Web apps are accessed through the web. They are platform-independent and are accessed via URL. Web apps can also function like mobile apps. This approach (Progressive Web Apps) allows developers to build a single version of an application for web and mobile platforms.
How to build a simple web application step-by-step
Building a simple web application involves 6 steps:
- Build the backend
- Set up your database
- Configure the middleware
- Build the frontend
- Host the web app
- Test and deploy

Build the backend
Programming a web app used to be complex and required knowledge of languages and hardware. Software frameworks significantly simplify development.
Creating the project and its architecture
To start building a web app with Ruby on Rails, for example, you use the command “rails new my_app” in your console or terminal. This creates a new web application called "my_app". You then use a scaffold command to create a scaffold that includes a model, views, and a controller for a specific database table.
Terminal Command
rails new my_app
Web application frameworks follow architectural patterns for web application development. In Rails, this pattern is model-view-controller, and in Django, it is Model-View-Template.
What’s Model View Controller (MVC)?
MVC is a model used to organize web applications. Unlike static web pages, web applications are a bit more complex and require a structure. MVC is a structure with the following components:
- Model: this is where the data, logic, and rules live.
- View: this is the user-facing part, where the user sees the data.
- Controller: The controller is an intermediary between the model and the view. It takes a user request and tells the Model what to do. It also chooses what to show to the user.
This structure keeps things organized. Generally, the model holds the data, and the view displays the data in different formats. For example, one view displays user posts and comments, and another shows the user profile they can edit. The controller makes sure the user sees what they requested and that the Model and View don’t have to talk directly to each other.
Creating application environments
Developers support several application environments. Here is a short introduction to each environment:
- The development environment is set up on the individual developer’s computer;
- The testing environment is used to test the application;
- The staging environment represents a copy of the production environment for final testing;
- The production environment is used to deploy the application to the production servers.
Backend frameworks might automatically set up some environments for you.
Setting up user authentication
This step usually includes:
- Planning the user flow or how users will log in, log out, and actions required when they forget their passwords
- Decide which user information to store, such as email, passwords, and names.
- Deciding on the user authentication method: Nowadays, OAuth 2.0 is a common standard. You can leverage third-party integrations such as Google and Facebook.
You also need to learn about legislation around how data is used in the app. The EU’s General Data Protection Regulation (GDPR) defines the European Union's privacy and data governance rules. In the US, consider the following legislation: Health Insurance Portability and Accountability Act, Family Educational Rights and Privacy Act, Children Online Privacy Protection Act, and California Consumer Privacy Act.
You can use cloud-based web services to offload typical security maintenance and ensure your web app is deployed with the latest security updates.
Building the backend is not a trivial task. If you are a beginner, try a tool like ondeva to build the backend logic and set up user authentication. The low-code platform simplifies development, so you can start immediately and focus on tasks other than backend building.

Set up your database
Next, you will design your database schema.
Designing a database schema involves designing the tables you want, determining columns for each table and their data types, planning the relationships between tables (one-to-many, many-to-many), considering how to index the tables, etc. The schema doesn’t contain the actual data. You could use tools like Dbeaver or MySQL Workbench to design the schema. These tools help you define the entity-relationship model and document your database schema.
Types of databases
Databases can be non-relational, relational, and schema-less. Relational databases are powerful tools for connecting data from different tables. For example, in a web application where users create posts, you can connect a table with user data to a table with posts via the author’s ID.

User Table
ID
Name
1
2
“Mary”
“James”

Post Table
ID
Text
Author
1
2
“Hello World”
“How to get started”
2
1
Infographic 1 - An example of relational database
Schema-less databases (NoSQL) don't require a predefined schema. NoSQL database houses data within one data structure, such as a JSON document. You can add new fields or data types without modifying existing data or database schema. NoSQL database doesn’t use SQL to query data but has its own methods. For example, MongoDB - a NoSQL database, offers query languages similar to SQL.
ondeva allows you to use schema-less databases. It enables you to create connections, upload datasets, and integrate data from multiple resources.
Database configuration and migrations
Next, you need to configure your database. The database is configured in the backend by specifying the database type (SQLite, MySQL, PostgreSQL, etc.) and connection credentials.
Once the database is configured, you can interact with it using Object-Relational Mapping without needing SQL.
The database needs to be migrated each time it is updated. For example, the database should be migrated if you need to add a column, delete a table, or add a relationship between different tables.
What is Object-Relational Mapping (ORM)?
The database is a collection of tables with data. The web browser uses HTML to show content, and the backend uses object-oriented language (Ruby on Rails, Python, etc.), so data is stored as objects on the application. The way data is stored in the database doesn’t always match the way data is stored as objects in the app. Object- Relational Mapping is used to account for these differences in data. In Ruby on Rails, for example, the ORM is called the Active Record Design Pattern. In Active Record, each table in the database has a corresponding class in the code. Records in the table are represented as instances of the class.
The database needs to be migrated each time it is updated. For example, the database should be migrated if you need to add a column, delete a table, or add a relationship between different tables.
Associations and Validations
It would be best if you also learn about associations and validations in the web app. Associations define the relationships between data (one-to-one, one-to-many, or many-to-many.). Validations are rules that are checked before data is entered into the database.
One to One
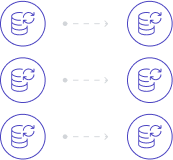
One to Many
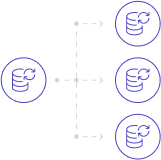
Many to Many
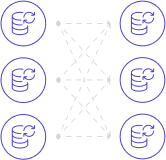
For example, you can ensure that a particular column is not empty, that a value in the form is in a number format or establish a mandatory password length. Each programming language or framework has its way of creating associations and validations.

Configure the middleware
Middleware services handle the code that connects the app to the web server.
The primary role of middleware is to provide the following services:
- Authentication: middleware checks if a user is authenticated before allowing access
- Logging every incoming request and outgoing response
- Session management: middleware handles session persistence between requests
- Error handling: middleware can catch and handle errors and return a response.
The web application framework usually provides middleware frameworks. For example, in Ruby on Rails, the middleware framework is called Rack. Some web app frameworks don’t offer middleware frameworks but features and components that achieve similar goals.
HTTP endpoints
In this stage, you need to configure HTTP endpoints for your frontend.
What’s the HTTP protocol?
The HTTP protocol delivers resources on the web through a response-and-request model, where the client sends an HTTP request to the server, and the server sends back the HTTP response. It is a stateless protocol, meaning each client request is independent and unrelated to previous requests. Therefore, HTTP cannot create the interactivity the users want, so other components were designed, such as cookies and sessions.
The client-side methods for HTTP requests include HEAD, GET, OPTIONS, TRACE, POST, PUT, AND DELETE.
Regarding HTTP responses, there are 5 status codes: 1xx—requires the requestor to take additional action, 2xx—request successfully processed, 3xx—redirected or further action is needed, 4xx—error request, and 5xx—internal server error when processing the request.
Sessions and cookies
Since HTTP is a stateless protocol that doesn’t remember the previous sessions, sessions, and cookies provide a way to retain user information. When the HTTP client sends a request and the server responds, the server response contains the session ID with the information related to the session. This session ID helps the server to refer to previously stored information about the user and respond accordingly. The session ID is usually sent to the browser as a cookie. The cookie is stored in the browser and sent back to the server with every request.
You must set up request and response processing in the backend using the chosen framework.
Creating routes (URLs)
Creating routes (URLs) for your application is typically designed following the RESTful (Representational State Transfer) architecture.
What are RESTful Web Services?
A RESTful Web Service is a type of service that adheres to the principles of REST (Representational State Transfer). REST is a philosophy that is built on the principle of stateless connections. The server in REST maintains a simple set of identifiable resources, which can be accessed through CRUD (Create, Read, Update, Delete) operations. The only operations you can apply to the vast number of available server resources are CRUD operations, so REST limits the operations, making it easier to write REST-based web applications. REST applications can be compared to RPC (Remote Procedure Call) applications. Unlike REST, in RPC-based web clients, the client sends the request to the server, and the server gives a response. For a client to know what to call for, the server and client need to agree on the procedure names and parameters, which allows for more flexibility and can be more specific than simple CRUD operations.

Build the frontend or presentation layer
Building the front end usually starts with understanding the basic principles of design, wireframing to create the basic structure of pages, and designing mockups that visually represent how the buttons, forms, and other UI elements will look. You can also hire a web designer to create mockups.
Alternatively, you can use ondeva AI builder for designing a page. You can build pages by visually dragging the elements and then asking AI to fill it with relevant content and images.
For building the frontend, the following technologies are used.
- HTML for creating the structure of web pages
- CSS for styling and visually formatting HTML elements
- JavaScript to add interactivity to elements
- Front-end frameworks and libraries to make it easier to program the frontend if you’re just starting.
HTML elements start with a tag and end with a tag, with content between them. HTML allows the assignation of attributes to elements such as ID and class elements. Styling rules through CSS and interactions through JavaScript are attached to HTML elements through IDs or classes. HTML requires the web page to have a standard structure with a DOCTYPE declaration, HTML tag, head, and body.
CSS (Cascading Style Sheets) creates rules for how particular HTML elements should appear. It uses selectors that specify the HTML element and a declaration that contains the properties and their values.
JavaScript allows for the creation of interactions and logic. You can also use JavaScript libraries, such as jQuery, which make programming with JavaScript more efficient.
Another technology you need to learn is Ajax (Asynchronous, JavaScript, XML).
What is Ajax?
Before Ajax, each time the client made a request, they had to wait until the response was received, so they could not interact with the browser. Ajax solved this problem. Ajax relies on JavaScript on the client side to respond to the request so the user can keep browsing. Ajax also employs XHR (XMLHttpRequest) or Fetch API, which allows web pages to update asynchronously by exchanging small amounts of data with the server without a full page reload.
You can also learn about dynamic content and accessibility. Dynamic content is personalized for the user and changes based on user interactions and preferences.
Accessibility laws differ from country to country. In the US, they are mandatory and can be enforced by an informal complaint. In Germany, the Disability Equality Act BGG dictates that federal government sites should be accessible. There is also EU accessibility legislation, such as the EU Accessibility Act 2025.

Host the web app
To host the app, you must buy a domain, purchase and set up an SSL certificate, and choose a cloud hosting provider, such as Amazon Web Services, GoDaddy, or Bluehost.
If you’re using any backend services, such as BaaS or PaaS, sometimes they provide servers to host your app, but you still need to buy a domain name and SSL certificate.
With ondeva, you need to buy a domain, which you can then set up in the ondeva domain settings.
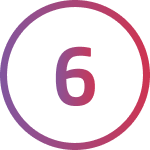
Test and deploy
You can deploy your app with tools such as Jenkins or Gitlab to test and deploy or deploy manually. To deploy manually, you will use an FTP/SFTP client to upload your application files to the server. You should also update DNS settings to tell the internet where to find your new app.
What will the web app cost you?
As a low-code platform built by developers, ondeva helps you focus on creative tasks.
Start for free. Pay only after 100 monthly page views.
Sign-up and get building in seconds - no payment, credit card, or demo required.
Traditional vs modern (low-code) development
Historically, web applications evolved from static web pages to full-scale web apps with interactive elements, cookies, more functionality on the client side, the ability to save the state while browsing, etc. The development of web applications was also once affected by the type of browser, but this is not the case anymore.
As web applications evolved, so did the development practices.

Traditional Development
In traditional development, you code everything from scratch using programming languages such as Ruby, Go, and Python for the backend and HTML, CSS, and JavaScript for the frontend. You also use SQL to build and model your database.

Modern Development (low-code)
No-code and low-code tools allow users to build apps from scratch, without coding. To use a low-code tool, one still needs to understand the main steps of web app building, business logic, how the database is constructed, etc.
Traditional development can be made more efficient in many ways. Language frameworks and database tools can increase the speed of development. Nowadays, it is rare to code an app from scratch.
Increasingly, developers use tools with a higher level of abstraction to create web apps. These are low-code/no-code tools coupled with AI.
Both low-code and no-code platforms simplify development.
Read here about low-code vs traditional development and how to choose the development approach.
Low-code app development with ondeva
As you can see, the traditional development of web apps can become complex. The learning curve is steep. With a low-code platform, you get a shorter learning curve but follow the same web app development process with no coding required. Some everyday development tasks are already automated for you; for example, you won’t have to load any frameworks or install database management tools. This makes the low-code platform perfect for beginner developers or projects requiring quick MVP prototyping.
How to use the low code platform

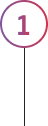
Step 1: Import your data
Add your tables to ondeva, then handle and structure the data.

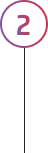
Step 2: Build your logic
Set rules for the way your application behaves on the backend and frontend.

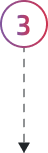
Step 3: Design your product
Use your own front-end stack or perfect the appearance of your application in ondeva.
Start building web apps with the low-code platform
Web app development is an essential process that every developer has experience with, but it takes work. Successfully creating a web app requires knowledge of front-end languages and frameworks, backend, database management, and some legislation on privacy and accessibility.
With ondeva, you can follow the same process but 10x times faster without needing to code. Our clients’ stories confirm the scalability and flexibility of low-code platforms for web development. If you’re a beginner developer, ondeva is your key to building web applications and optimizing developer workflows so you can focus on more hard-to-solve problems or creative tasks.
FAQ
The cost of web application development varies. It depends on the app's complexity, the features required, and the technology stack used. Typically, fees range from 5,000 for simple applications to over 250,000 for complex ones. To get a more accurate cost estimate, prepare a specification document outlining all the features and expectations and describe the app's appearance. Alternatively, you can hire a developer and work alongside them to create the specification document. You can also learn web development, but the costs for web hosting, cloud computing services, domain names, and user interactions (such as email services and SMS) will come separately.
Scalability is the ability of the web application to adapt to an increased load. Building a scalable web application involves planning technology and architecture, including a scalable hosting solution, such as cloud services, the microservices architecture that ensures different application components can be scaled independently, and a database that supports horizontal scaling.
Web application security practices should be integrated throughout the development lifecycle. These practices include following secure coding practices to prevent SQL injections and XSS (Cross-Site Scripting), using multi-factor authentication, and using HTTPS and TLS to encrypt data in transit. Additionally, educate yourself on the latest web security threats and best practices. You can find detailed web app security checklists online.
If you want to develop a serverless web app, you should first look for a cloud provider with a solution offering serverless capability. Serverless capability or architecture allows users to write and run their code in response to events and other triggers. At that point, you will have to decide what the architecture of your application will be and create stateless functions for its various processes like processing data, logging in a user, etc. The cloud provider will then deploy those functions as you configure event triggers to call them.
The steps to create a web app include:
- Setting up the development environment
- Building the backend
- Setting up your database
- Configuring the middleware
- Building the frontend
- Testing and deploying
Before this process, you usually have an idea of the application and the specifications of features, appearance, and expectations. After this process, you likely need ongoing maintenance, improving performance following user feedback, and a marketing plan to acquire new users.